Tutorial: Setting up the password change functionality with Django
In the original Hello Web App book, I walk you through the process of setting up your registration and login pages so user's can join and log into your new app. We also set up the password reset views on the login form, so if a user doesn't remember their login information, they can get a reset link emailed to them.
We also set up the templates for the password change functionality, so logged in users can update their password, but we didn't set up the URLs and templates in order to get that working. Let's do that here!
Set up the URL
We already set up the URLs for password reset functionality — adding the password change functionality is very similar:
urls.py
# add to the top
from django.contrib.auth.views import (
password_reset,
password_reset_done,
password_reset_confirm,
password_reset_complete,
# these are the two new imports
password_change,
password_change_done,
)
urlpatterns = [
# the previously added URL definitions
url(r'^accounts/password/reset/done/$',
password_reset_done,
{'template_name': 'registration/password_reset_done.html'},
name="password_reset_done"),
# new url definitions
url(r'^accounts/password/change/$', password_change, {
'template_name': 'registration/password_change_form.html'},
name='password_change'),
url(r'^accounts/password/change/done/$', password_change_done,
{'template_name': 'registration/password_change_done.html'},
name='password_change_done'),
We're going to have two new pages on our website, one that allows for changing the password for a logged-in user, and a success page after successful reset.
In our import statement, we import django-registration-redux views for password_change
and password_change_done
, and then we tie those two the new URLs we're adding, linking to the templates we already set up in the original tutorial.
Add a link in the templates
The last thing we need to do is provide a link to these new pages from our app. I'm putting this in the same page where the user updates their object, but it could be put anywhere in your app:
edit_thing.html
<!-- add me somewhere in your app -->
<a href="{% url 'password_change' %}">
Change password
</a>
We know what URL to link to as we named it password_change
in urls.py. Open up the page in your browser and you should see the new form:
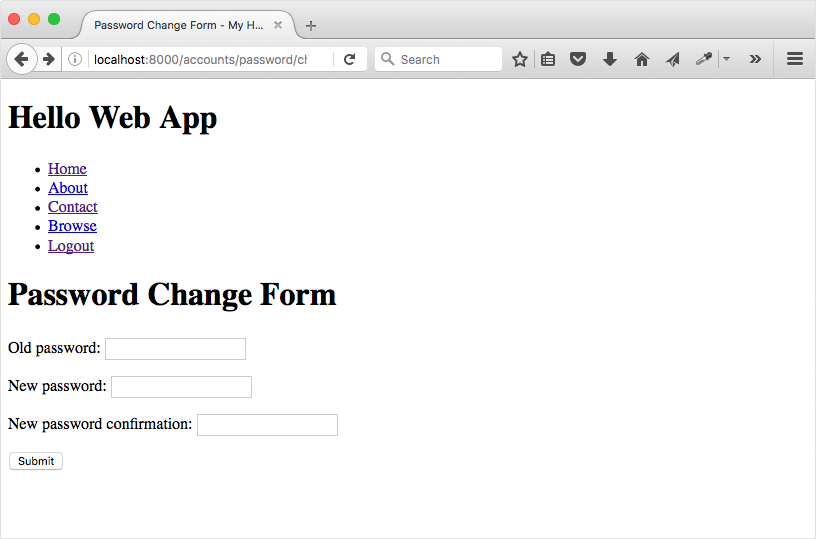
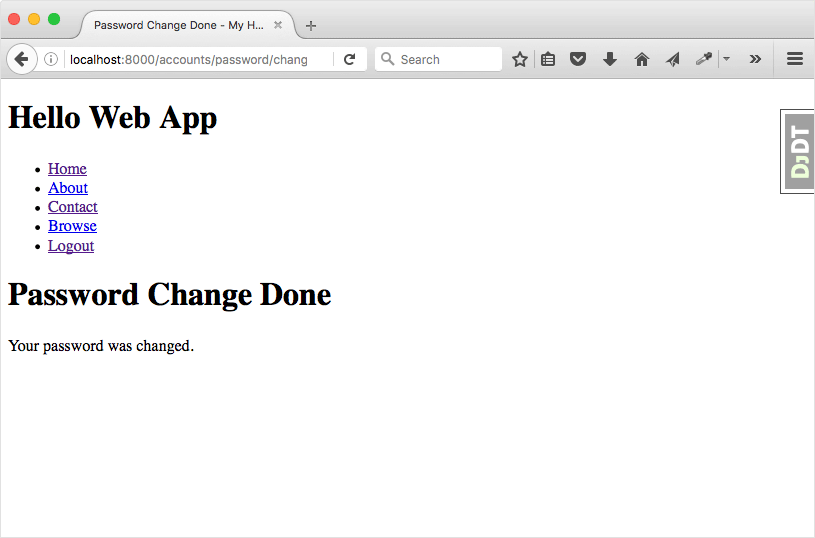
Try testing it out with one of your test users, and logging in and out using the new passwords. Everything should be working swimmingly. That's all you need to do to get the password change functionality working. Congrats!
This tutorial is meant as an add-on to my book, Hello Web App, teaching beginner web app development using Django. If you haven't taken a look at it yet, check it out and buy here: Hello Web App
Thanks everyone, and happy to answer any questions in the comments!